newbie server / client best practice question
Options
Hello All,
I am relatively new to both Unity and photon. I have completed a few of the unity tutorials and wanted to try making a simple multiplayer game. I figured I would do a multiplayer version of roll a ball where a few players connect, collect all the objects, then you win.
I started playing around with the DemoRPGMovement scene.
I created a cylinder, made it a prefab then added the "PhotonView" and "PhotonTransformView" scripts that are attached to the "Robot Kyle RPG" prefab that comes with the demo. I then added the following lines to 'DemoRPGMovement' script in the 'server' build
void CreatePickupObjects()
{
Vector3 cubePosition = new Vector3 (50, 5, 62);
GameObject newSphere = PhotonNetwork.Instantiate ("Prefab1", cubePosition, Quaternion.identity, 0);
}
for the 'client' build, I removed these lines but kept the prefab.
My question is, is it mandatory for the Cylinder prefab to be in the client build so that the connecting clients can see the prefab? ( I tried making a client build without the prefab however the that client was not able to see the cylinder I am assuming the prefab needs to be loaded somehow from the server if it is not in the client build) Or would this be bad practice similar to calculating player move speed in the client which would lead to exploitation? Obviously this does not matter when I am just learning / playing around with the demo, but I was just curious from a best practice perspective if we should keep models in client builds, or if I should keep the client to a bare minimum and research loading the prefab from the server once the client is connected (If this is the case, would anyone be able to point me to a few tutorials or keywords that I can google to find a good example?)
If my post is confusing, I uploaded a quick video to youtube.
I ran my server out of unity, and I had 2 exe's one with the prefab in the client, and one without.
https://www.youtube.com/watch?v=xRPSylM3LoI
I am relatively new to both Unity and photon. I have completed a few of the unity tutorials and wanted to try making a simple multiplayer game. I figured I would do a multiplayer version of roll a ball where a few players connect, collect all the objects, then you win.
I started playing around with the DemoRPGMovement scene.
I created a cylinder, made it a prefab then added the "PhotonView" and "PhotonTransformView" scripts that are attached to the "Robot Kyle RPG" prefab that comes with the demo. I then added the following lines to 'DemoRPGMovement' script in the 'server' build
void CreatePickupObjects()
{
Vector3 cubePosition = new Vector3 (50, 5, 62);
GameObject newSphere = PhotonNetwork.Instantiate ("Prefab1", cubePosition, Quaternion.identity, 0);
}
for the 'client' build, I removed these lines but kept the prefab.
My question is, is it mandatory for the Cylinder prefab to be in the client build so that the connecting clients can see the prefab? ( I tried making a client build without the prefab however the that client was not able to see the cylinder I am assuming the prefab needs to be loaded somehow from the server if it is not in the client build) Or would this be bad practice similar to calculating player move speed in the client which would lead to exploitation? Obviously this does not matter when I am just learning / playing around with the demo, but I was just curious from a best practice perspective if we should keep models in client builds, or if I should keep the client to a bare minimum and research loading the prefab from the server once the client is connected (If this is the case, would anyone be able to point me to a few tutorials or keywords that I can google to find a good example?)
If my post is confusing, I uploaded a quick video to youtube.
I ran my server out of unity, and I had 2 exe's one with the prefab in the client, and one without.
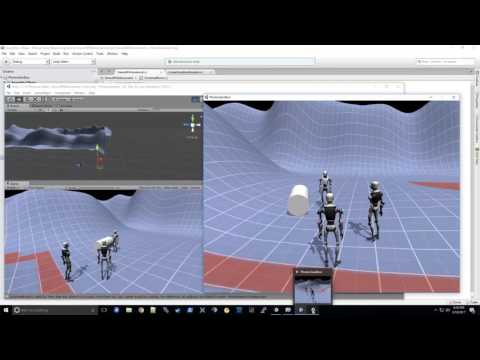
0
Comments
-
Hi @mcegamesmobile,My question is, is it mandatory for the Cylinder prefab to be in the client build so that the connecting clients can see the prefab?
in short: yes. More detailed: When using PhotonNetwork.Instantiate(...) it raises an event internally that is sent across the network to notify other clients to do the same. If you have removed the prefab before building the client, the client receives this raised event and throws an error message because the prefab could not be found. You probably didn't see this error message because you may have not build a development build. When building the game you can check that option to receive error messages on screen.
Keep in mind that the prefab itself is not sent across the network.0 -
Thank you Christian!
one more question if you have the time. What about terrain? The client builds also have a copy of the map terrain in them. Should the terrain only be in the server build and have that data sent to the client instead of having the terrain in the client build in order to prevent say, having the client add a random ramp / ladder to my map if they wanted to cheat in my game?0 -
Hi @mcegamesmobile,
cheating in multiplayer games is always a big problem and you can't prevent users from cheating. In my opinion it is impossible, since people will always find a way to gain unfair advantages.
I guess by 'server build' you mean the MasterClient. You should include the terrain in the build (for all clients) and do not try to send the terrain data somehow to other users across the network. Keep in mind that when you are using the Cloud you don't have any kind of server instance. If you however need a server instance, you need to check and host a Photon Server by yourself. This can be extended with plugins and let's you inject custom logic on server side (however I guess this would be too much right now).0