How do I make the ball physics?
Options
Hi everyone, I'm very sorry for that my English is very bad.
Actually problem is obvious from the picture. I don't want to serialize the position of the ball. For this, serialize the velocity I did but I couldn't. Anyway, my English is not so good. I hope you know that.
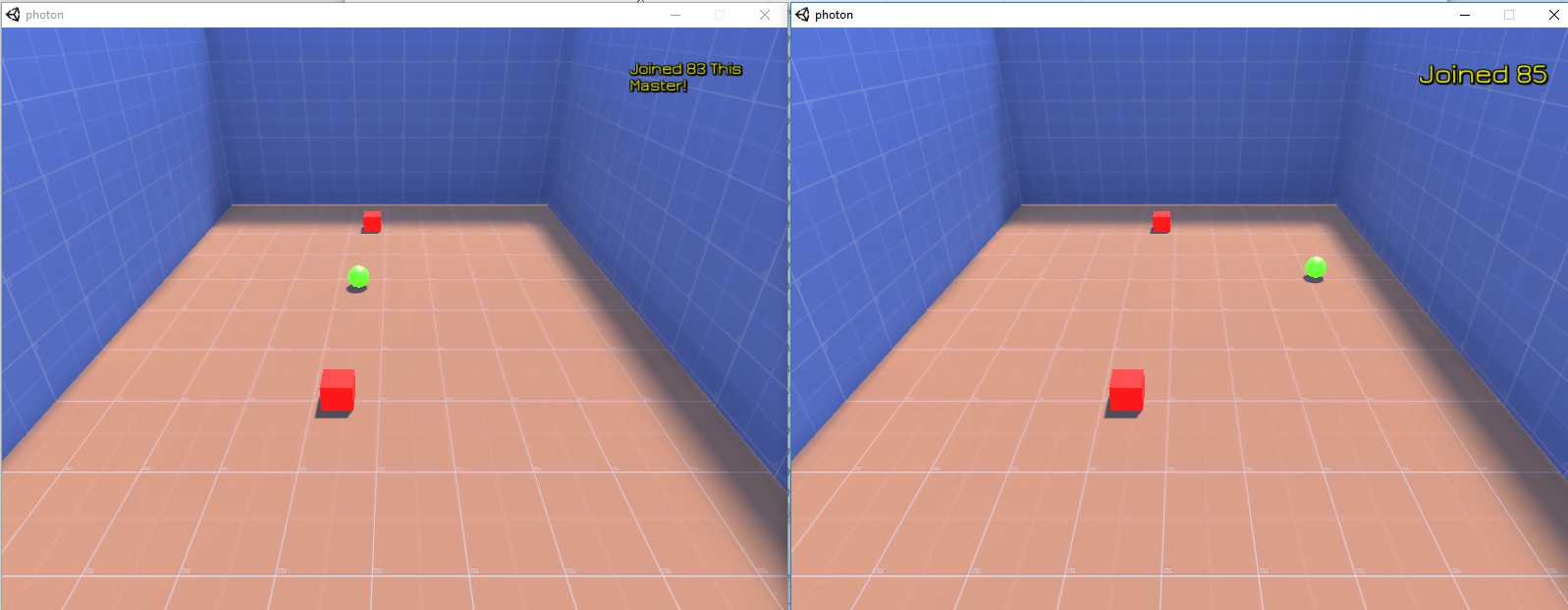
Code:
Other objects of the code(both players)
Actually problem is obvious from the picture. I don't want to serialize the position of the ball. For this, serialize the velocity I did but I couldn't. Anyway, my English is not so good. I hope you know that.
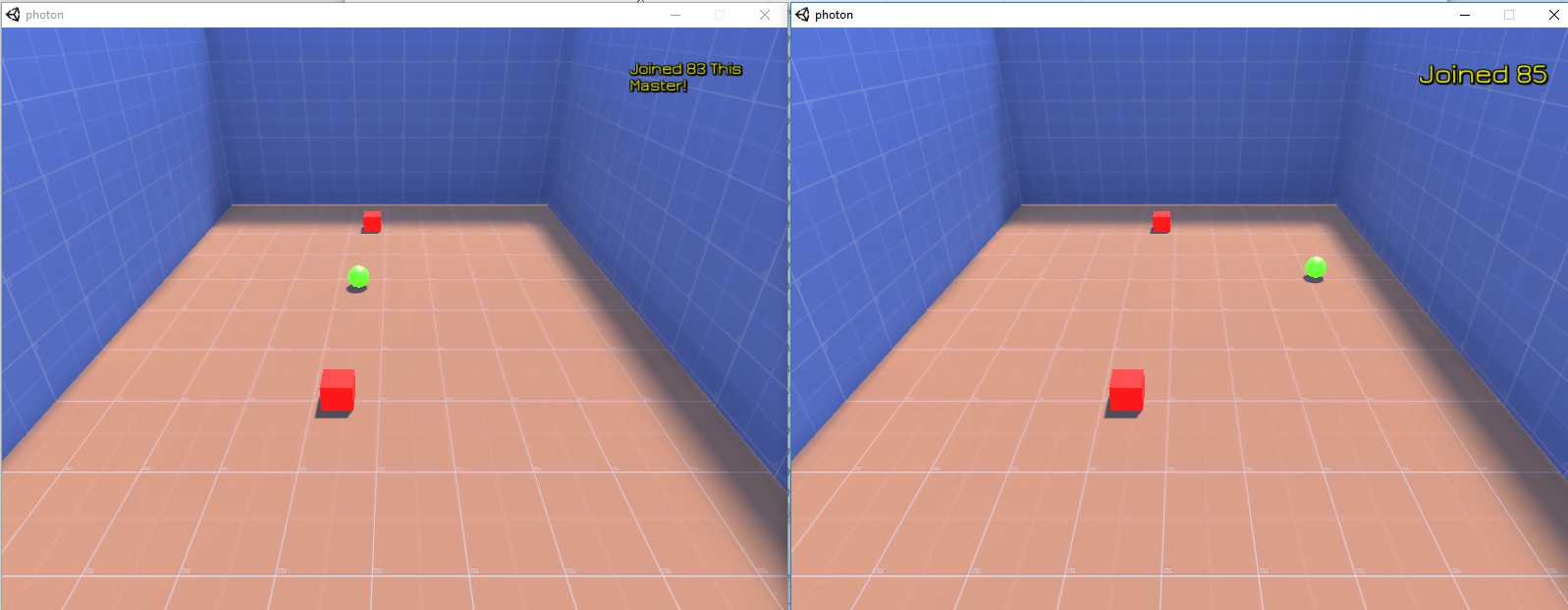
Code:
Other objects of the code(both players)
using UnityEngine;
using System.Collections;
using Photon;
public class myrigidbody : Photon.MonoBehaviour {
Vector3 prevPosition;
public Vector3 velocity;
public float velocityMultiple;
GameObject ball;
void Start() {
PhotonNetwork.sendRate = 60;
PhotonNetwork.sendRateOnSerialize = 60;
Application.targetFrameRate = 60;
}
void Update() {
velocity = ((transform.position - prevPosition) / Time.deltaTime) * velocityMultiple;
prevPosition = transform.position;
}
void OnCollisionEnter(Collision coll) {
if (coll.gameObject.name == "ball") {
if (ball == null)
{
ball = coll.gameObject;
}
photonView.RPC("shoot", PhotonTargets.All, velocity);
}
}
void OnPhotonSerializeView(PhotonStream stream, PhotonMessageInfo info)
{
if (stream.isWriting)
{
stream.SendNext(velocity.x);
stream.SendNext(velocity.z);
}
else
{
velocity.x = (float)stream.ReceiveNext();
velocity.z = (float)stream.ReceiveNext();
}
}
[PunRPC]
public void shoot(Vector3 velocityy)
{
ball.GetComponent<Rigidbody>().velocity += velocityy;
}
}
0
Comments
-
Hi,
You set 'velocity' property twice, in Update() and in OnPhotonSerializeView(). Most likely you want to do so only once. To restrict updates to local objects only in Update() method, use photonView.isMine property.0