Rooms List/Map Selection/Rotation
Options
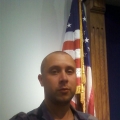
N1warhead
✭✭
Hey guys, okay, I've been sitting here sense 7 AM, eastern time USA. And I can't figure nothing out when it comes to Lobbies, Rooms, etc.
I can get it to play online with two people +, no problems there.
But I can't seem to find ANYTHING, in the documentation or anywhere on how to set up a GUI System for room selection.
I DID however, copy the code off the Worker demo and got it to load my level show it in the Roomlists, etc.
However, I'm not even sure if I did it right or not. I just merely tried it, it loaded my level, but now I'm not sure if it messed up my ingame code or anything like that.
E.G. - I changed my in-game code to get my player(s) to spawn, but now getting a nullreference error (once again).
Then that gets to my MAIN QUESTIONS,
I don't know ANYTHING about what a hashtable is, where to put it, how to put it, what to put it in, or anything.
And don't see how that allows the person wanting to host a room to select a map, game mode, etc.
And to allow users to filter what game modes they want to do.
The little documentation there is makes it seem as if it's hardly any code and that the Hashtable thing is what does it.
But again, doesn't say where to apply it too, do I put it in Update, Start, Awake, FixedUpdate, etc.
So how is any of this done?
Here is my Player Instantiate script (Maybe you can help me find the Nullreference Error when two people or more join server
And for quick access, here is your Workers Demo script for the rooms list, maybe I can get some pointers to what to do and where to place something at.
Please help me!
THANK YOU!!
I can get it to play online with two people +, no problems there.
But I can't seem to find ANYTHING, in the documentation or anywhere on how to set up a GUI System for room selection.
I DID however, copy the code off the Worker demo and got it to load my level show it in the Roomlists, etc.
However, I'm not even sure if I did it right or not. I just merely tried it, it loaded my level, but now I'm not sure if it messed up my ingame code or anything like that.
E.G. - I changed my in-game code to get my player(s) to spawn, but now getting a nullreference error (once again).
Then that gets to my MAIN QUESTIONS,
I don't know ANYTHING about what a hashtable is, where to put it, how to put it, what to put it in, or anything.
And don't see how that allows the person wanting to host a room to select a map, game mode, etc.
And to allow users to filter what game modes they want to do.
The little documentation there is makes it seem as if it's hardly any code and that the Hashtable thing is what does it.
But again, doesn't say where to apply it too, do I put it in Update, Start, Awake, FixedUpdate, etc.
So how is any of this done?
Here is my Player Instantiate script (Maybe you can help me find the Nullreference Error when two people or more join server
public class PlayerInstantiate : MonoBehaviour { // THIS SCRIPT IS REQUIRED ON EVERY SINGLE LEVEL!!! // THIS IS TO DEAL WITH INSTANTIATING CHARACTERS // FOR SPAWN SPOTS ON JOINING THE ROOM!!!!! // // MAKE SURE THERE ARE SPAWN SPOTS ON EVERY MAP WITH THE // SPAWN SPOTS SCRIPT ATTACHED TO THEM! // OR IT WILL NOT WORK. public GameObject standbyCamera; // This is for the standby camera, when player dies, it's a camera to wait to respawn again. SpawnSpot[] spawnSpots; // This searches the Array for spawnSpots. //Use this for initialization void Start () { // finds the spawns on the execution of the script! spawnSpots = GameObject.FindObjectsOfType<SpawnSpot> (); } void Connect(){ // if server doesn't have the exact "Toony Wars V1.0" or 1.1, 1.2, etc they cant join the same game. PhotonNetwork.ConnectUsingSettings (" Toony Wars V1.0"); // EVERY GAME UPDATE CCHANGE THIS NUMBER!!!! (ALLLOWS ONLY PEOPLE WITH SAME UPDATE TO PLAY) } void OnJoinedRoom(){ SpawnMyPlayer (); Debug.Log("Connected to Room"); } void SpawnMyPlayer(){ if (spawnSpots == null) { Debug.LogError ("No Spawn Spots in map! PUT SPAWNS IN MAP"); return; } //Spawns the player SpawnSpot mySpawnSpot = spawnSpots [Random.Range (0, spawnSpots.Length)]; GameObject myPlayerGO = (GameObject)PhotonNetwork.Instantiate("PlayerController", mySpawnSpot.transform.position, mySpawnSpot.transform.rotation, 0); standbyCamera.SetActive(false); //((MonoBehaviour)myPlayerGO.GetComponent("FPSInputController")).enabled = true; //((MonoBehaviour)myPlayerGO.GetComponent("CharacterMotor")).enabled = true; ((MonoBehaviour)myPlayerGO.GetComponent("MouseLook")).enabled = true; ((MonoBehaviour)myPlayerGO.GetComponent("PlayerMovement")).enabled = true; myPlayerGO.transform.FindChild ("Main Camera").gameObject.SetActive (true); } }
And for quick access, here is your Workers Demo script for the rooms list, maybe I can get some pointers to what to do and where to place something at.
using System; using UnityEngine; using Random = UnityEngine.Random; public class NetworkManager : MonoBehaviour { private string roomName = "myRoom"; private Vector2 scrollPos = Vector2.zero; private bool connectFailed = false; public static readonly string SceneNameMenu = "MainMenu"; public static readonly string SceneNameGame = "Deserta"; private string errorDialog; private double timeToClearDialog; public string ErrorDialog { get { return errorDialog; } private set { errorDialog = value; if (!string.IsNullOrEmpty(value)) { timeToClearDialog = Time.time + 4.0f; } } } public void Awake() { // this makes sure we can use PhotonNetwork.LoadLevel() on the master client and all clients in the same room sync their level automatically PhotonNetwork.automaticallySyncScene = true; // the following line checks if this client was just created (and not yet online). if so, we connect if (PhotonNetwork.connectionStateDetailed == PeerState.PeerCreated) { // Connect to the photon master-server. We use the settings saved in PhotonServerSettings (a .asset file in this project) PhotonNetwork.ConnectUsingSettings (" Toony Wars V1.0"); } // generate a name for this player, if none is assigned yet if (String.IsNullOrEmpty(PhotonNetwork.playerName)) { PhotonNetwork.playerName = "Guest" + Random.Range(1, 9999); } // if you wanted more debug out, turn this on: // PhotonNetwork.logLevel = NetworkLogLevel.Full; } public void OnGUI() { if (!PhotonNetwork.connected) { if (PhotonNetwork.connecting) { GUILayout.Label("Connecting to: " + PhotonNetwork.ServerAddress); } else { GUILayout.Label("Not connected. Check console output. Detailed connection state: " + PhotonNetwork.connectionStateDetailed + " Server: " + PhotonNetwork.ServerAddress); } if (this.connectFailed) { GUILayout.Label("Connection failed. Check setup and use Setup Wizard to fix configuration."); GUILayout.Label(String.Format("Server: {0}", new object[] {PhotonNetwork.ServerAddress})); GUILayout.Label("AppId: " + PhotonNetwork.PhotonServerSettings.AppID); if (GUILayout.Button("Try Again", GUILayout.Width(100))) { this.connectFailed = false; PhotonNetwork.ConnectUsingSettings("0.9"); } } return; } GUI.skin.box.fontStyle = FontStyle.Bold; GUI.Box(new Rect((Screen.width - 400) / 2, (Screen.height - 350) / 2, 400, 300), "Join or Create a Room"); GUILayout.BeginArea(new Rect((Screen.width - 400) / 2, (Screen.height - 350) / 2, 400, 300)); GUILayout.Space(25); // Player name GUILayout.BeginHorizontal(); GUILayout.Label("Player name:", GUILayout.Width(100)); PhotonNetwork.playerName = GUILayout.TextField(PhotonNetwork.playerName); GUILayout.Space(105); if (GUI.changed) { // Save name PlayerPrefs.SetString("playerName", PhotonNetwork.playerName); } GUILayout.EndHorizontal(); GUILayout.Space(15); // Join room by title GUILayout.BeginHorizontal(); GUILayout.Label("Roomname:", GUILayout.Width(100)); this.roomName = GUILayout.TextField(this.roomName); if (GUILayout.Button("Create Room", GUILayout.Width(100))) { PhotonNetwork.CreateRoom(this.roomName, new RoomOptions() { maxPlayers = 10 }, null); } GUILayout.EndHorizontal(); // Create a room (fails if exist!) GUILayout.BeginHorizontal(); GUILayout.FlexibleSpace(); //this.roomName = GUILayout.TextField(this.roomName); if (GUILayout.Button("Join Room", GUILayout.Width(100))) { PhotonNetwork.JoinRoom(this.roomName); } GUILayout.EndHorizontal(); if (!string.IsNullOrEmpty(this.ErrorDialog)) { GUILayout.Label(this.ErrorDialog); if (timeToClearDialog < Time.time) { timeToClearDialog = 0; this.ErrorDialog = ""; } } GUILayout.Space(15); // Join random room GUILayout.BeginHorizontal(); GUILayout.Label(PhotonNetwork.countOfPlayers + " users are online in " + PhotonNetwork.countOfRooms + " rooms."); GUILayout.FlexibleSpace(); if (GUILayout.Button("Join Random", GUILayout.Width(100))) { PhotonNetwork.JoinRandomRoom(); } GUILayout.EndHorizontal(); GUILayout.Space(15); if (PhotonNetwork.GetRoomList().Length == 0) { GUILayout.Label("Currently no games are available."); GUILayout.Label("Rooms will be listed here, when they become available."); } else { GUILayout.Label(PhotonNetwork.GetRoomList() + " currently available. Join either:"); // Room listing: simply call GetRoomList: no need to fetch/poll whatever! this.scrollPos = GUILayout.BeginScrollView(this.scrollPos); foreach (RoomInfo roomInfo in PhotonNetwork.GetRoomList()) { GUILayout.BeginHorizontal(); GUILayout.Label(roomInfo.name + " " + roomInfo.playerCount + "/" + roomInfo.maxPlayers); if (GUILayout.Button("Join")) { PhotonNetwork.JoinRoom(roomInfo.name); } GUILayout.EndHorizontal(); } GUILayout.EndScrollView(); } GUILayout.EndArea(); } // We have two options here: we either joined(by title, list or random) or created a room. public void OnJoinedRoom() { Debug.Log("OnJoinedRoom"); } public void OnPhotonCreateRoomFailed() { this.ErrorDialog = "Error: Can't create room (room name maybe already used)."; Debug.Log("OnPhotonCreateRoomFailed got called. This can happen if the room exists (even if not visible). Try another room name."); } public void OnPhotonJoinRoomFailed() { this.ErrorDialog = "Error: Can't join room (full or unknown room name)."; Debug.Log("OnPhotonJoinRoomFailed got called. This can happen if the room is not existing or full or closed."); } public void OnPhotonRandomJoinFailed() { this.ErrorDialog = "Error: Can't join random room (none found)."; Debug.Log("OnPhotonRandomJoinFailed got called. Happens if no room is available (or all full or invisible or closed). JoinrRandom filter-options can limit available rooms."); } public void OnCreatedRoom() { Debug.Log("OnCreatedRoom"); PhotonNetwork.LoadLevel(SceneNameGame); } public void OnDisconnectedFromPhoton() { Debug.Log("Disconnected from Photon."); } public void OnFailedToConnectToPhoton(object parameters) { this.connectFailed = true; Debug.Log("OnFailedToConnectToPhoton. StatusCode: " + parameters + " ServerAddress: " + PhotonNetwork.networkingPeer.ServerAddress); } }
Please help me!
THANK YOU!!
0
Comments
-
Hi
It's not quite clear what you are trying to do and what does not work for you. If I missed some of your questions, please repeat it.
When client is in lobby it can get list of available rooms with PhotonNetwork.GetRoomList(). The method returns array of RoomInfo's with all room infos including room properties (map, game mode?) marked as 'listed in lobby' during room creation. Use that list for building room selection menu in OnGUI() method.
For analyzing 'Nullreference Error', full error message with call stack required.0 -
Thank you for your reply back Vadim.
Sorry I didn't make my self very clear on my questions, sorry.
What I am trying to do is -
(1) - When player starts game, choose between single and multiplayer (THIS IS DONE)
(2) - BUT, when the player hits multiplayer, give them a choice between random matchmaking,
OR finding a room to join or hosting a game.
(3) - if someone wants to host a game, how do I make a Gui show a list of available maps the user can select, same with game mode (Death Match, Team Death Match)
(3 continued) - If user hosts a room, do I make it a whole new script for dealing with Ingame stuff, or do I keep everything on one Network Manager script?
I think what is getting me the most is the GUI Systems, how do I make it show another GUI once I click Multiplayer, to give like 3 more buttons (quick match, host, join).
Sorry if I am asking for so much, I love how good Photon works, Photon isn't the problem, it's me.
I got so used to Unityscript, I am having to re-learn C# all over again. I heard I could use UnityScript with Photon,
but then again, there is almost 0 help when it comes to doing this with Unityscript, so I am re-learning C#.
Because you see, every time I start trying to figure things out, I end up breaking an entire game and not able to fix it.
I would never have thought making a menu was harder than making any other aspect of a game.
I've had to start over on a game twice just this week alone.0 -
I think you need some gui 'state' variable to draw ui elements in OnGUI() depending on current state (with switch statement).
Change state when user chooses multiplayer, hosting game or other action.
Follow Unity GUI scripting guide http://docs.unity3d.com/Manual/gui-Basics.html for more information on gui drawing.If user hosts a room, do I make it a whole new script for dealing with Ingame stuff, or do I keep everything on one Network Manager script?0 -
Thank you for your help, I'll look into this......
I love Photon, just having to re-learn C# and how certain things work.
When I started with Unity I started with C# but quickly began to realize,
95% of all tutorials were in Unityscript, so I converted to US, slowly getting the hang of C# again lol.0